JavaScript 高级
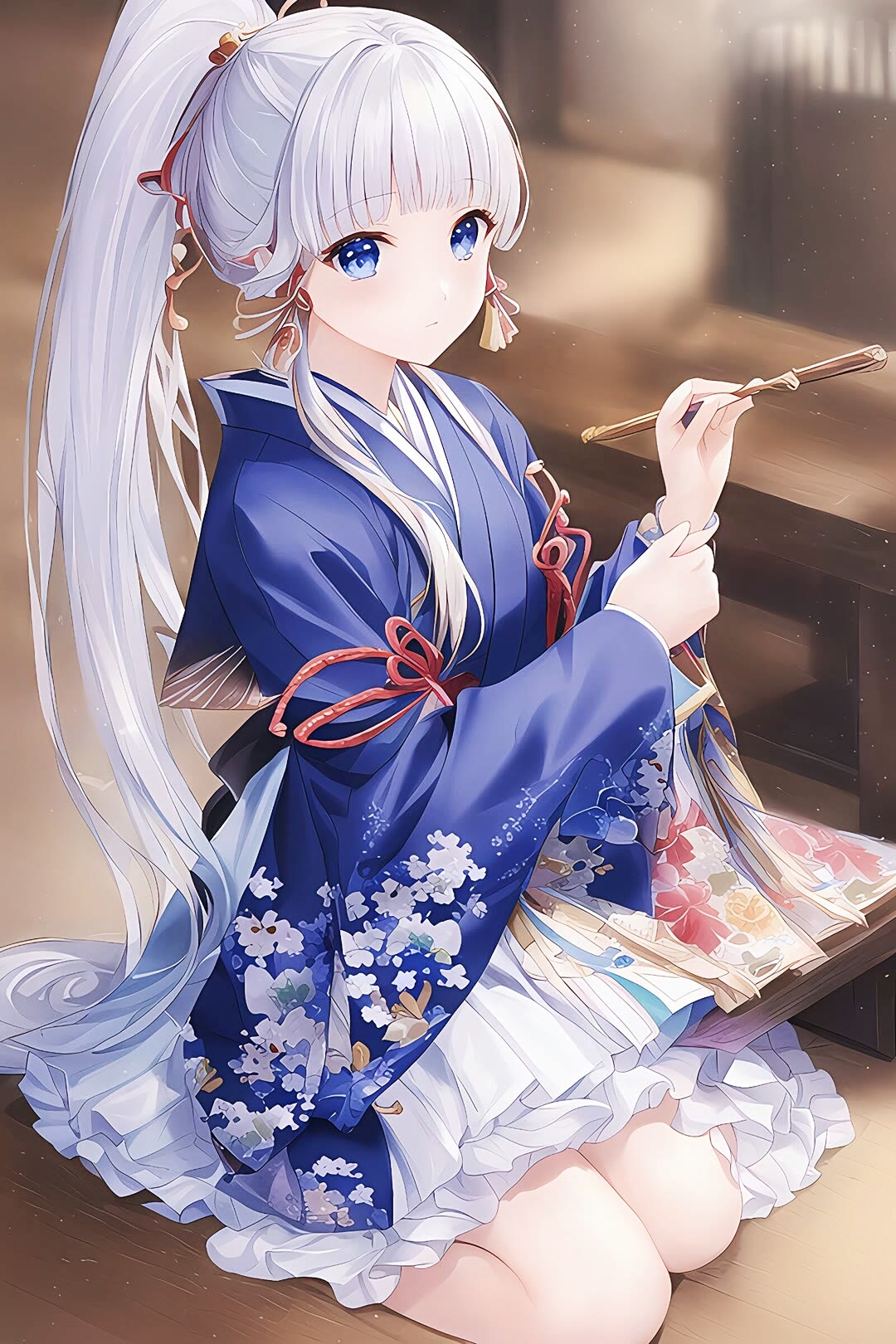
JavaScript 高级
WaterBoat01.call, apply 和 bind 的区别
call 的使用方式:
1 | //#region call |
apply 的使用方式:
1 | //#region apply |
bind 的使用方式:
1 | //#region bind |
总结
- call,apply 和 bind 都有改变 this 指向的作用
- call 和 apply 的区别不过是 call() 方法接受的是一个参数列表,apply() 方法接受的是一个包含多个参数的数组
- bind 与其他两个的区别是不会立即执行,会返回一个函数在需要的时候再执行